My name is Jin Ying, a Year 2 Student reading Computer Science at National University of Singapore. This project portfolio documents my contributions to the project.
Overview
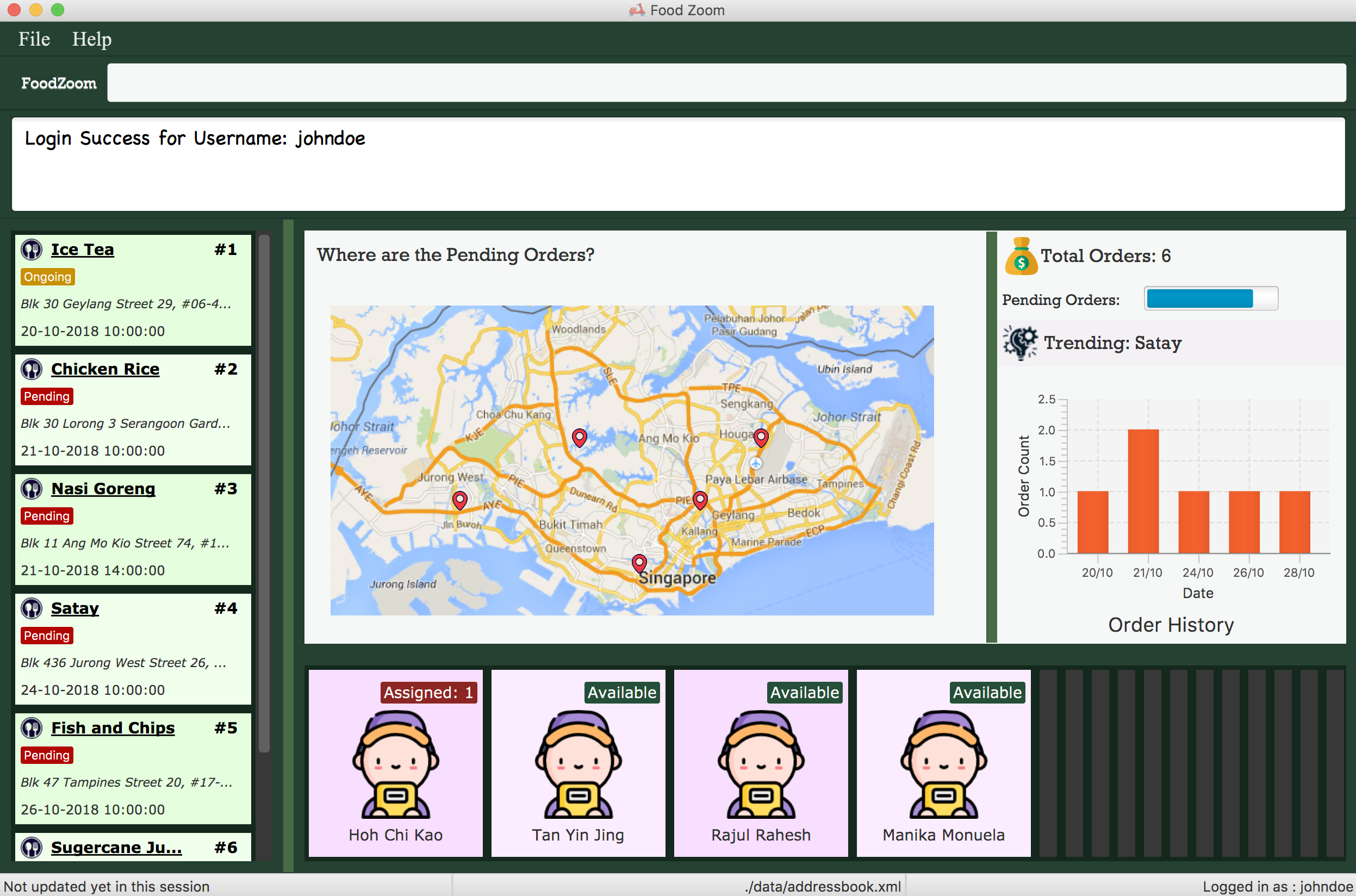
FoodZoom is a desktop application providing food businesses a delivery management platform for them to streamline their delivery process. FoodZooms' responsiveness and quickness allows manager to plan for route through a Command Line Interface (CLI). The platform also allows delivery man to view their delivery routes and increase their efficiency. FoodZoom is created by 5 students, namely Tan Jin Ying, Monika Manuela, Julius Sander, Rahul Rajesh and Koh Chi Hao.
Summary of Contribution
-
Major enhancement:
-
Added the ability to find for existing order/s
-
What it does: It allows the user to find for existing order in the list of orders with any order parameter that it was created with.
-
Justification: This feature improve the ease for the user to look for the order in the potential long list of order they could have.
-
Highlights: The enhancement changes the
INDEX
in the User Interface. Any command that relies on theINDEX
needs keep this in mind.
-
-
Added the ability to edit existing order
-
What it does: This feature allows the user to make amendments to any order they have created previous.
-
Justification: As a user could add the order with the wrong parameter, this feature allows the user to make any edits to the order.
-
Highlights: This enhancement creates a new
Order
object and any future commands that need a reference to the originalOrder
object need to be able to track a change in the object.
-
-
-
Minor enhancement: added a find command to search for existing deliveryman
-
Code Contribution: RepoSense
-
Other contributions:
Contributions to User Guide
This section shows my contributions to User Guide for the project. It showcases my ability to write user-centric documentation. |
Finding orders : /order find
[Since v1.2]
Find any order/s with any given order fields.
Format: /order find [n/NAME] [p/PHONE] [a/ADDRESS] [f/FOOD] [dt/DATETIME] [st/ORDER_STATUS]
Examples:
-
/order find n/john
Returnsjohn
andJohn Doe
-
/order find p/81231233 a/block 123, Clementi Drive, #01-01
Returns orders with phone number of81231233
and address ofblock 123, Clementi Drive, #01-01
-
/order find dt/01-10-2018 10:00:00 dt/03-10-2018 10:00:00
Returns for orders that are within the datetime01-10-2018 10:00:00 and 03-10-2018 10:00:00
Editing an order : /order edit
[Since v1.2]
Edits an existing order in the list of orders.
Format: /order edit INDEX [f/FOOD] [n/NAME] [p/PHONE] [a/ADDRESS] [dt/DATETIME]
Examples:
-
/order list
/order edit 1 p/91234567 n/Jonathan
Edits the phone number and name of the 1st order to be91234567
andJonathan
respectively. -
/order list
/order edit 2 f/Maggi Goreng f/Ice Milo
Edits the food of the 2nd order to beMaggi Goreng, Ice Milo
.
Finding delivery man by name : /deliveryman find
[Since v1.2]
Find delivery men whose name contain in the given parameter.
Format: /deliveryman find n/NAME
Examples:
-
/deliveryman find n/John Smith
Returns all delivery men with the namesJohn
e.g.John Smith
andJohn Doe
Contributions to Developer Guide
This section shows my contributions to Developer Guide for the project. It showcases my ability to write a technical document and the technical aspect of my contribution to the project. |
Find Order Feature
Current Implementation
The order find
command allows searching of orders in FoodZoom. It allows searching for orders with any order fields.
The following sequence diagram shows the sequence flow from the LogicManager
to the ModelManager
when a user enter a find
command:
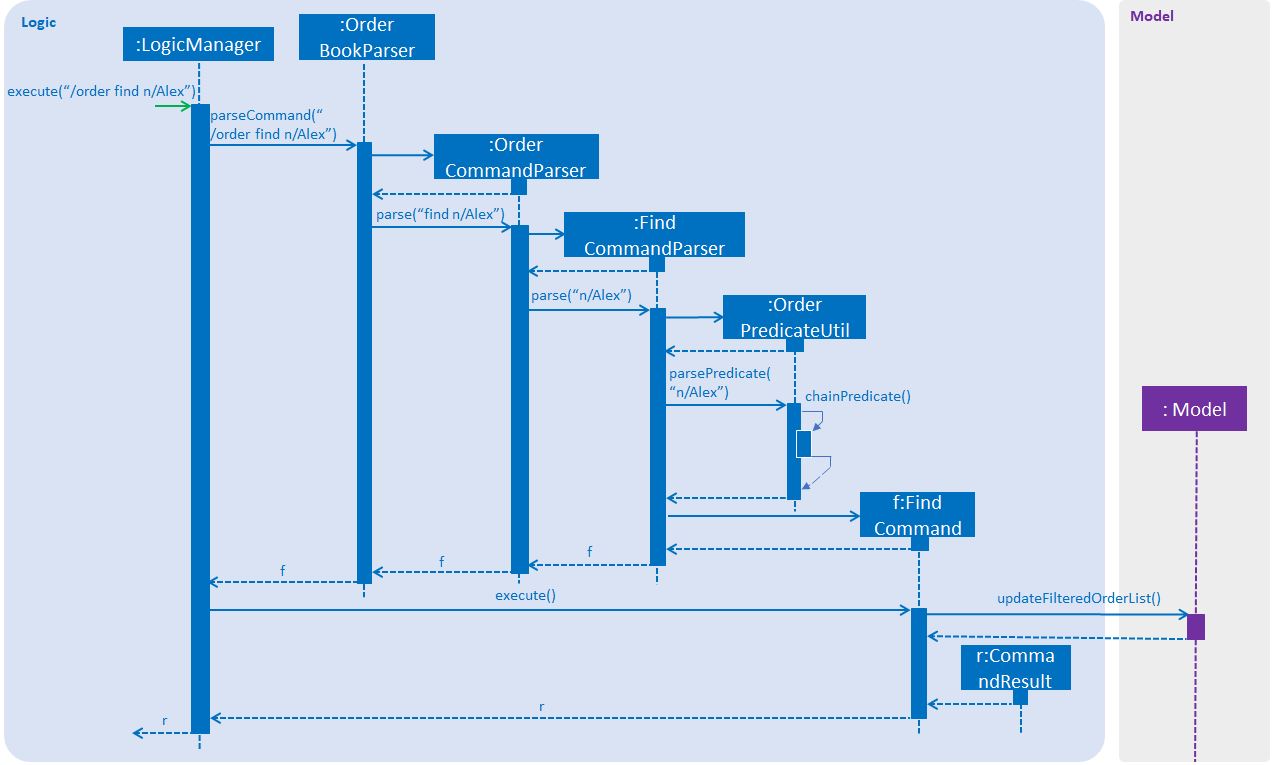
Figure 3.4.1.1 Sequence Diagram for order find
command
From the sequence diagram:
-
When
LogicManager
receive theexecute
command, it calls theparseCommand
method inOrderBookParser
. -
OrderBookParser
will receive/order
as the command and instantiateOrderCommandParser
to further parse the command. -
OrderCommandParser
receivefind
as the command and callsFindCommandParser
to handle the fields for the find command. -
If at least one field is provided,
FindCommandParser
will callparsePredicate
ofOrderPredicateUtil
to handle the given fields. -
OrderPredicateUtil
will call its ownchainPredicate
to create the predicate for the relevant fields. -
If all fields give are valid,
FindCommand
will be created and return back toLogicManager
. -
LogicManager
will proceed to call execute ofFindCommand
. -
FindCommand
will update the list in theModel
and create a newCommandResult
to be returned.
The differentiation is handled by OrderPredicateUtil
which checks for the fields provided. It throws ParseException
if a invalid order field is specified or when any field is given without any value.
OrderPredicateUtil
will chain up the different fields and return a Predicate<Order>
to narrow the scope
of the search.
Datetime
search can be performed in two ways. Below is a sequence diagram to show flow of how datetime
search:
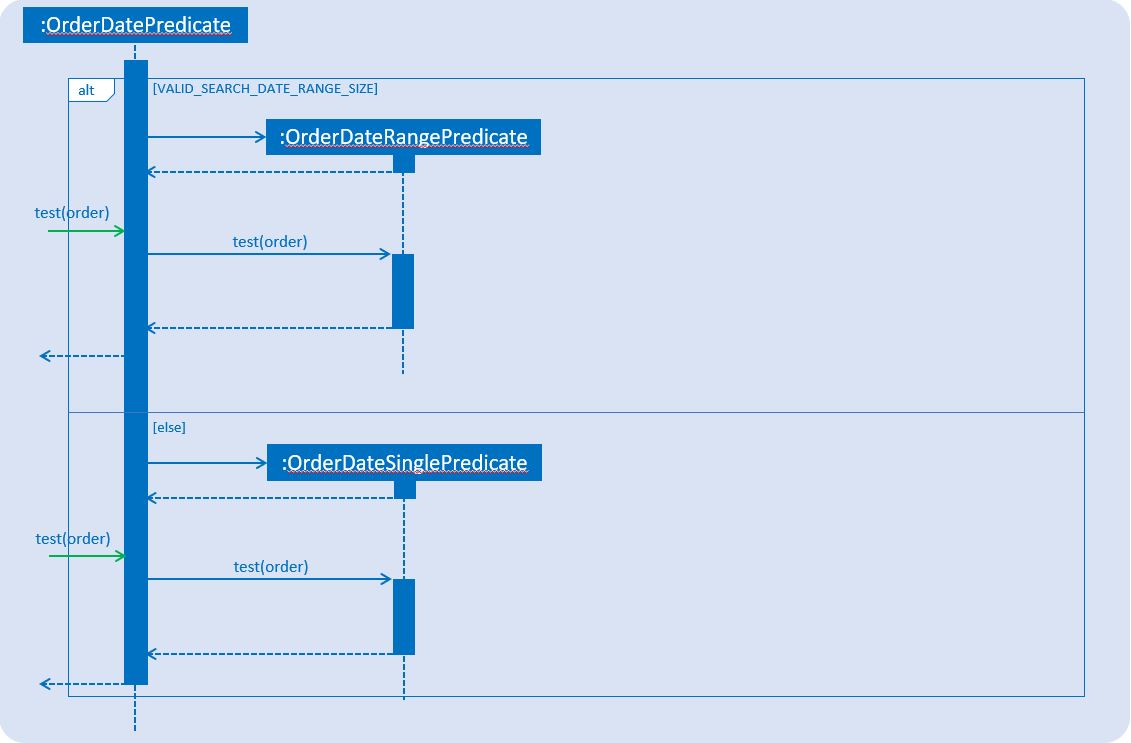
Figure 3.4.1.2 Sequence Diagram for datetime
search
-
If one
datetime
field is given,OrderDatePredicate
creates aOrderDateSinglePredicate
object and run its'test
method. -
If more than one
datetime
field is given,OrderDatePredicate
creates aOrderDateRangePredicate
object and run its'test
method.
Design Consideration
Aspect: Implementation of FindCommand
-
Alternative 1 (current choice): All different order field having its own test method.
-
Pros: It adheres to the Single Responsibility Principle (SRP) and the Separation of Concern (SoC). Each predicate handles its own search values.
-
Cons: More classes will be needed, which results in higher complexity of the code base.
-
-
Alternative 2: One predicate class to handle all the predicates.
-
Pros: Reduces the number of classes, which makes it for new developers to understand.
-
Cons: It decreases the cohesion as one class will need to handle different predicates.
-
Aspect: Implementation of FindCommandParser
-
Alternative 1 (current choice): Further parsing of the predicates are passed to
OrderPredicateUtil
to handle-
Pros: It adheres to SRP as the handling of the predicates are passed on to
OrderPredicateUtil
class. -
Cons: Increase in the complexity of the code as more classes are needed, with more lines of code being written
-
-
Alternative 2:
FindCommandParser
to handles the predicates.-
Pros: It makes it easy for new developers to understand the code as
FindCommandParser
handles all the parsing of the predicates -
Cons: It violates SRP.
FindCommandParser
has to break down the fields as well as check which fields are present.
-